使用範例:
<p id = 'clover'>Lucky clover 🍀</p>
Inline styles
直覺簡單的方法,但不適用於有太多元素都要改CSS時使用。
document.querySelector('#clover').style.color = 'darkgreen';
或是使用Element.style.cssText
,可以自己增加裡面的屬性。
document.querySelector('#clover').style.cssText = 'color : darkgreen';
Global styles
先使用createElement()
創建一個style
元素,再透過innerHTML
新增style
裡面的值,最後用document.head.appendChild()
將style
新增到head
裡。
let style = document.createElement('style');
style.innerHTML = `#clover {color: darkgreen;}`;
document.head.appendChild(style);
classList
以上方法都可以透過JS來修改CSS,不過這邊有更好的方法來管理、修改CSS語法,那就是使用classList
。
classList提供了許多methods來幫助我們更新、移除CSS語法:
item(number)
- 找查class的indexcontains(string)
- 檢查此class有無存在,返回布林值add(string [,string])
- 新增classremover(string [,string])
- 移除classtoggle(string [, force])
- 切換class
使用classList之前我們要先建立CSS的樣式表以做新增或移除...等:
.darkgreen {
color: darkgreen;
}
.bold {
font-weight: 900;
}
.decoration {
text-decoration: overline 1px blue;
}
新增class:classList.add()
新增'darkgreen'與'bold'兩個class:
let clover = document.querySelector('p');
clover.classList.add('darkgreen', 'bold');
移除class:classList.remover()
移除bold class:
clover.classList.remove('bold');
切換class:classList.toggle()
toggle(string [, force])
有兩個參數,如果只有第一個參數,則語法會去檢查這個class是否存在,如果存在則移除這個class,並返回false。反之,此class不存在的話,則新增此class並返回true:
clover.classList.toggle('darkgreen');
clover.classList.toggle('bold');
toggle的第二個參數是布林值,如果有第二參數是true的話則添加此class,如果第二參數設置為false則移除此class:
clover.classList.toggle('decoration', true);
clover.classList.toggle('bold', false);
document.style與window.getComputedStyle(el)的差異
在講差異之前我們先小小複習一下CSS樣式,等等會用到以下的範例講解差異:
Inline Styles
在tag中添加attribute
<p id = 'inlineStyle' style = 'color: green'>Inline Styles</p>
Internal Style Sheet
在<head>
之間添加<style>標籤
<head>
<style>
#internalStyleSheet{color: blue}
</style>
</head>
<body>
<p id = 'internalStyleSheet'>Internal Style Sheet</p>
</body>
External Style Sheet
外接link連結CSS樣式表
<head>
<link rel="stylesheet" href="externalStyleSheet
.css">
</head>
<body>
<p id = 'externalStyleSheet'>External Style Sheet</p>
</body>
//以下為外接CSS樣式表的內容:
#externalStyleSheet{
color:red}
我們將以上三種形式的CSS結合再一起後,測試看看el.style與window.getComputedStyle(el)的不同:
使用el.style:
//先select
let inline = document.querySelector('#inlineStyles');
let internal = document.querySelector('#internalStyleSheet');
let external = document.querySelector('#externalStyleSheet');
inline.style;
color的值顯示green
internal.style;
color的值是空的
external.style;
color的值是空的
2. window.getComputedStyle(el):
都有回傳color的rgb值
試試看直接用window.getComputedStyle(el)
改CSS樣式,console告訴我們window.getComputedStyle
唯讀無法修改:
總結
以上例子總結可以發現:
el.style
回傳的物件CSSStyleDeclaration
,只會顯示Inline Style形式的CSS,表示el.style
只能讀取Inline Style。window.getComputedStyle(el)
讀取的是最終樣式,包括Inline Style、Internal Style Sheet與External Style Sheet。- window.getComputedStyle(el)只能用來讀取樣式。
el.style
雖然只能讀取Inline Style,但可以修改CSS樣式,要注意的是它修改的地方是在tag中再加入style: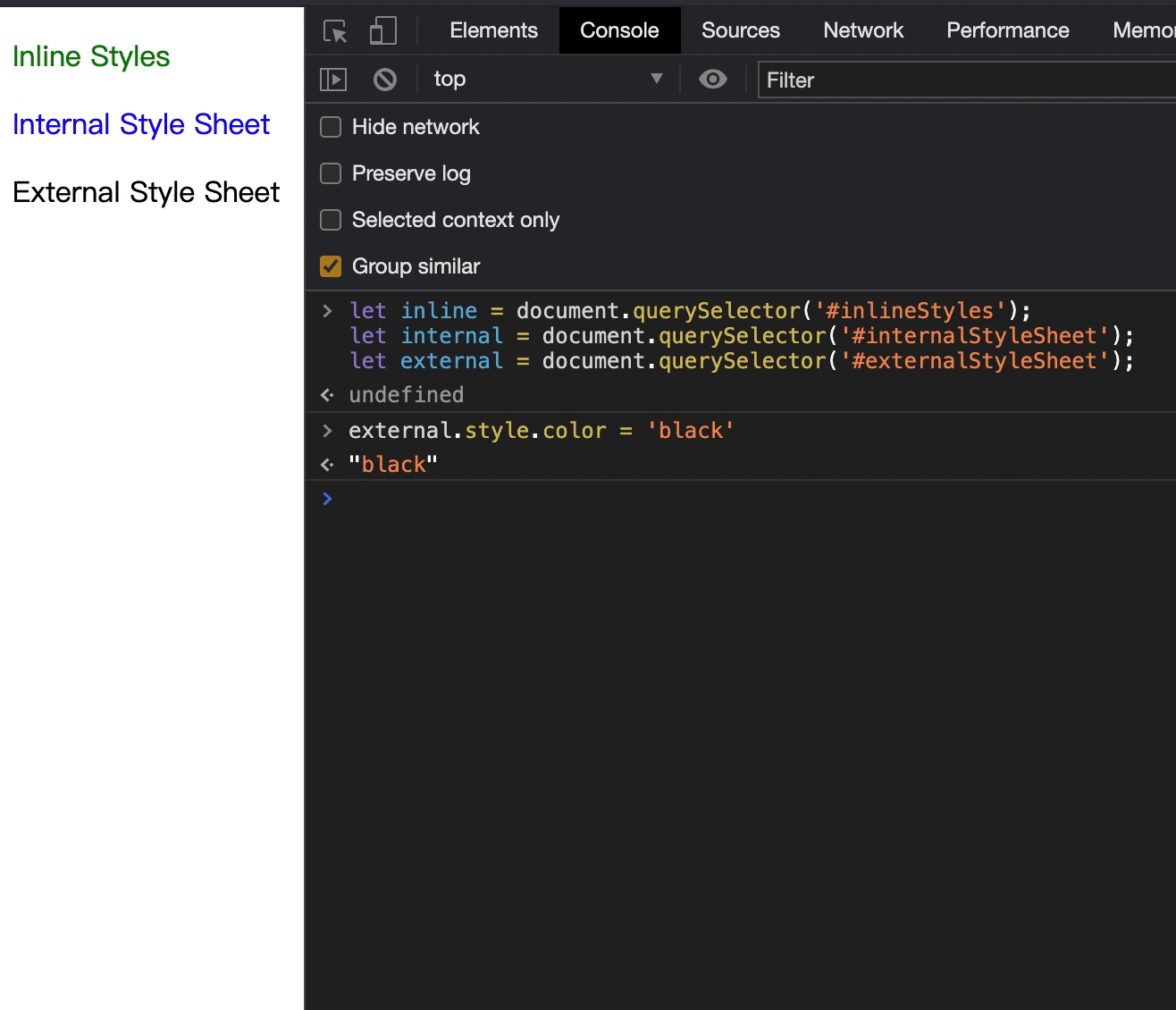
參考資料